Yolov4中使用了Mosica数据增强方法,能够在有限数据集情况下极大程度的增加增强样本量。本文对Mosica数据增强方法进行Python代码实现介绍
数据准备
本实现需要以下相关数据和文件准备
VOC数据集(本实验使用的是VOC2007)
classes文件
将VOC数据集预先经过处理后,得到一个合并所有标注信息的文件’annotations.txt’,其中每一行是图像绝对路径和BoundingBox和标签信息
1 | VOCdevkit/VOC2007/JPEGImages/000005.jpg 263,211,324,339,8 165,264,253,372,8 5,244,67,374,8 241,194,295,299,8 277,186,312,220,8 |
导入依赖包
1 | import random |
random用于生成随机数,以进行随机参数的变换
numpy用于图像和numpy的转换以及数据处理
Image用于图像加载和变换
ImageDraw和ImageFont用于在图像上画BoundingBox
pyplot用于绘图显示图像
定义一些工具函数
1 | # 用于生成指定范围的随机数 |
1 | # 用于从classes文件得到classes数组 |
1 | # 在图像上绘制box矩形框以及标签文字 |
图像样本显示
1 | # 变量预先设置 |
Mosica数据增强是以4副图像合并为一副图像,首先获取4副图像进行显示
1 | plt.figure(figsize=(16, 12)) |
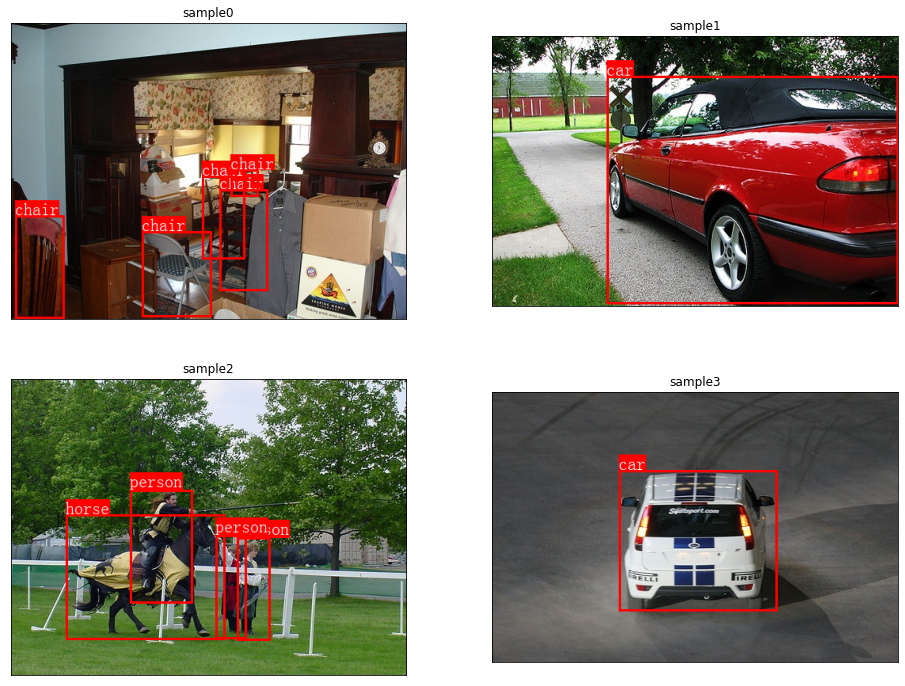
Mosica处理
1 | index = 0 |
box合并处理的函数
1 | def merge_bboxes(bboxes, cutx, cuty): |
将4副样本进行随机翻转、尺度变换后放置在背景图中的效果
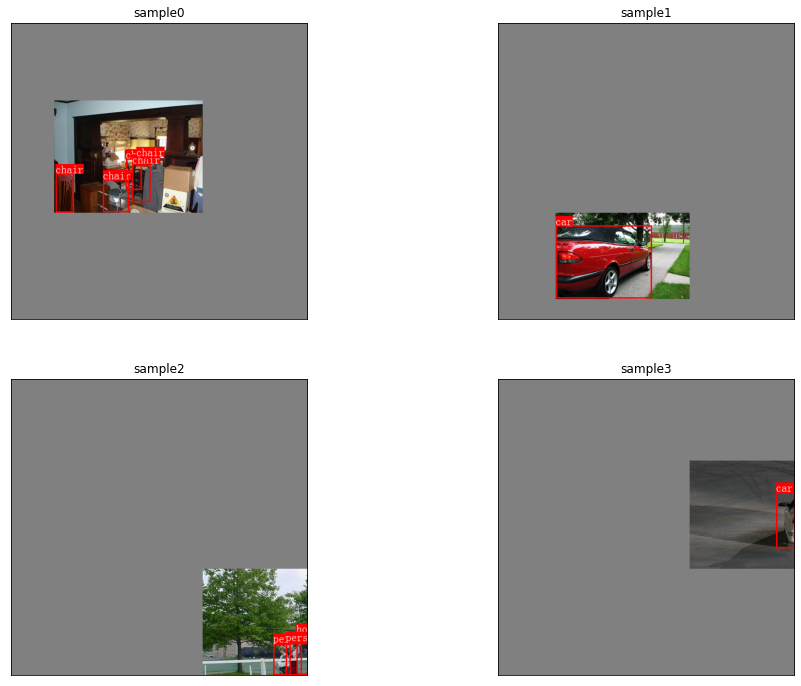
最终合成图的效果
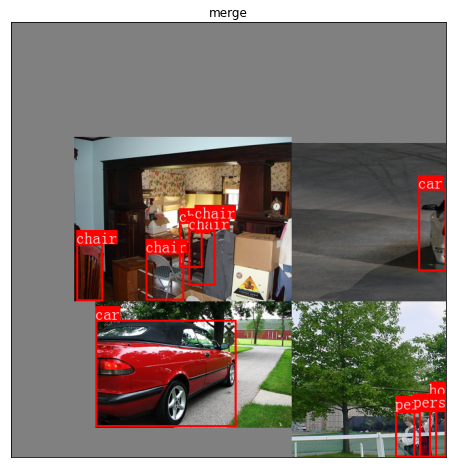